JavaScript Interview Questions and Answers: Welcome to the ultimate guide for JavaScript enthusiasts and aspiring developers! Whether you’re gearing up for your first job interview or looking to level up your JavaScript game, this post is your go-to resource. In the fast-paced world of tech interviews, being well-prepared is the key to success.
We’ve curated a comprehensive list of 100+ commonly asked JavaScript Interview Questions and Answers that will not only test your knowledge This post is your ticket to mastering JavaScript interviews with confidence. Let’s dive in and unravel the secrets to acing your JavaScript interviews together!
Basic JavaScript Interview Questions and Answers for Freshers (2024)
1. What is JavaScript
JavaScript is a lightweight, cross-platform, and very powerful high-level programming language that is commonly used to make web pages more interactive and dynamic. It allows developers to create responsive and attractive websites by adding features like form validation, animations, and interactive elements. It is also known as a scripting language for web pages.
2. What are datatypes in JavaScript?
JavaScript has several datatypes, which can be broadly categorized into two main types: primitive and object datatypes (non-primitive).
1. Primitive Datatypes:
- Number
- String
- Boolean
- Null
- Undefined
- Symbol
- BigInt
2. Object Datatypes (Non-primitive):
- Object
- Array
- Function
3. What are the differences between Java and JavaScript?
Java | JavaScript |
Java is a general-purpose, high-level programming language. | JavaScript is a scripting language primarily used for web development. |
It is both compiled and statically typed, requiring a compilation step before execution. | It is interpreted and dynamically typed, with the code executed line by line in a browser. |
Java code is typically run on a Java Virtual Machine (JVM), making it platform-independent. | JavaScript is run in web browsers and can manipulate the Document Object Model (DOM) to create dynamic content. |
Commonly used for server-side development, desktop applications, and Android mobile app development | Mainly used for client-side web development but also employed on the server-side (Node.js). |
Java is an object-oriented programming (OOPS) or structured programming language like C++ or C and .NET | JavaScript supports both OOP and procedural programming styles. |
4. Explain the difference between ‘undefined’ and ‘null’.
- Undefined is a primitive value automatically assigned to variables that have been declared but not initialized. It indicates that a variable has been declared but has no assigned value.
- Null is also a primitive value that represents the intentional absence of any object value. It is often used to explicitly indicate that a variable or object property should have no value.
5. How do you declare variables in JavaScript?
Variables can be declared using var, let, or const. While var has function-level scope, let and const have block-level scope. const is used for variables that should not be reassigned.
6. Explain the difference between let, const, and var.
- let and const have block-level scope, meaning they are limited to the block or statement where they are defined.
- const is used for variables that should not be reassigned after initialization.
- var has function-level scope and is less preferable due to its behavior during hoisting.
7. Explain Hoisting in JavaScript.
Hoisting is a default behavior in JavaScript where variable and function declarations are moved to the top of their containing scope during the compilation phase, before the code is executed. This means that you can use variables and functions in your code even before they are declared.
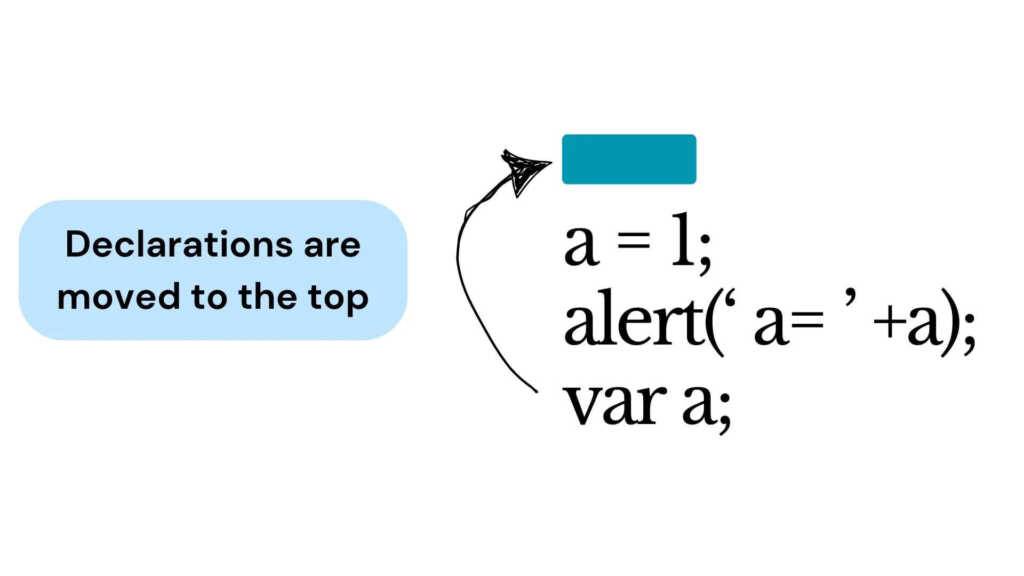
Note: Only the declarations are hoisted, not the initializations.
Hoisting with Variables:
console.log(x); // Outputs: undefined
var x = 5;
console.log(x); // Outputs: 5
JavaScriptIn this example, the declaration ‘var x;’ is hoisted to the top, so no errors occur in the first ‘console.log(x)’, but the value is ‘undefined’ until the actual assignment.
JavaScript moves the variable declaration to the top of its scope during the compilation phase. So, when you log x before its actual declaration, it doesn’t result in an error.
However, the value is undefined at that point because initialization (=5) is not hoisted. The second console.log(x) correctly outputs the initial value, which is 5.
Hoisting with Functions
sayHello(); // Outputs: "Hello, World!"
function sayHello() {
console.log("Hello, World!");
}
JavaScriptHere, the entire function declaration is hoisted, so you can call the function before its actual declaration in the code.
Note: In terms of variables and constants, the keyword ‘var’ is hoisted and ‘let’ and ‘const’ or with function expressions do not allow hoisting.
console.log(y); // Throws an error: Cannot access 'y' before initialization
let y = 10;
JavaScriptHere, the ‘let‘ variable is hoisted, but accessing it before the declaration results in an error due to the temporal dead zone (TDZ).
8. What is Temporal Dead Zone?
TDZ is a specific period in the execution of JavaScript code where variables declared with let and const exist but cannot be accessed or assigned a value. During this phase, accessing or using the variable will generate a Reference Error.
9. What are arrays, functions and objects?
Arrays: An array is like a container that holds a list of items. These items can be of any data type, such as numbers, strings, objects, and even other arrays. Array provide an organized way to group related elements, making it convenient to work with collections of data.
Each element in an array is assigned a unique index, starting from zero, which can be used to access or modify the values. Arrays are versatile and widely used in JavaScript for tasks like storing multiple values in a single variable, managing data, and performing various operations on sets of values.
Example:
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits[1]); // Output: 'banana'
JavaScriptFunctions: Functions in JavaScript are reusable blocks of code that perform specific tasks. They allow you to organize your code into modular pieces, making it easier to understand and maintain. Functions can take input, known as parameters, perform operations, and optionally return a result.
They can be declared using the ‘function’ keyword. Functions play a key role in structuring code, promoting reusability and encapsulating logic, making JavaScript program more efficient and maintainable.
Example:
function add(a, b) {
return a + b;
}
JavaScriptObjects: Objects act like containers that allow us to organize and store related information. They consist of key-value pairs, where each key is like a label pointing to a specific value.
Example:
let person = { name: "John", age: 30, occupation: "Developer" };
console.log(person.name); // Output: John
console.log(person.age); // Output: 30
console.log(person.occupation); // Output: Developer
JavaScript10. What is the purpose of the ‘typeof
‘ operator?
The JavaScript ‘typeof’ operator is used to determine the data type of a variable or expression. It returns a string representing the data type.
For Example:
typeof "Hello" //Returns "string"
typeof false // Returns "boolean"
typeof [1,2,3,4] // Returns "object"
typeof {name:'John', age:34} // Returns "object"
JavaScript11. What is ‘this’ Keyword in JavaScript?
In JavaScript, the ‘this‘ keyword refers to the current execution context, or the object to which the current code belongs. The value of ‘this‘ is determined by how a function is called. Unlike most programming languages, the value of ‘this‘ in JavaScript is not determined by where a function is declared, but by how it is invoked.
Example:
Let’s consider a simple example using this keyword in JavaScript within a method of an object.
// Define a Person object constructor
function Person(name, age) {
this.name = name;
this.age = age;
// Method to introduce the person
this.introduce = function() {
console.log("Hi, I'm " + this.name + " and I'm " + this.age + " years old.");
};
}
// Create instances of the Person object
const person1 = new Person("Alice", 28);
const person2 = new Person("Bob", 35);
// Call the introduce method for each person
person1.introduce(); // Outputs: "Hi, I'm Alice and I'm 28 years old."
person2.introduce(); // Outputs: "Hi, I'm Bob and I'm 35 years old."
JavaScriptIn this example:
- The Person constructor creates an instance with the properties name and age.
- The introduce method uses this keyword to refer to the current instance. It accesses the name and age properties of the specific Person object calling the method.
- When we create two instances (Person1 and Person2) and call the Introduce method for each, this keyword ensures that the correct properties are accessed, allowing each person to introduce themselves accurately.
12. How do you check if a variable is an array?
To check if a variable is an array in JavaScript, you can use the Array.isArray() method.
Here’s an example:
const myVariable = [1, 2, 3];
if (Array.isArray(myVariable)) {
console.log('It is an array!');
} else {
console.log('It is not an array.');
}
JavaScript13. Explain the event delegation in JavaScript.
Event delegation is a powerful technique in JavaScript that optimizes event handling by utilizing the concept of event bubbling. Instead of attaching individual event listeners to multiple similar elements, we attach a single event listener to a common ancestor of those elements, often a parent element or the document itself.
14. Explain Global Variables in JavaScript?
If the variable can be accessed anywhere in the our program is called global variables and these will not have any scope in the applications.
15. How do you prevent modification of an object in JavaScript?
In JavaScript, you can prevent modification of an object using Object.freeze() method. The Object.freeze() method freezes an object, preventing new properties from being added to it, existing properties from being removed, and preventing property values from being changed.
Here’s an example:
const myObject = {
property1: 'value1',
property2: 'value2'
};
// Freeze the object
Object.freeze(myObject);
// Attempt to modify the object
myObject.property1 = 'new value'; // This modification will not take effect
// Attempt to add a new property
myObject.property3 = 'value3'; // This addition will not take effect
// Attempt to delete a property
delete myObject.property2; // This deletion will not take effect
console.log(myObject); // Output: { property1: 'value1', property2: 'value2' }
JavaScriptIn this example, after calling Object.freeze(myObject), any attempts to modify, add, or delete properties on myObject are ignored, and the object remains unchanged. Object.freeze() creates an immutable version of the object, making it read-only.
16. Explain the difference between ‘==’ and ‘===’.
Both ‘==’ and ‘===’ are comparison operators. The difference is that ‘==’ compares values, while ‘===’ compares both values and types.
Example:
const num = 5;
const str = '5';
console.log(num == str); // true
console.log(num === str); // false
JavaScript17. What is a callback function?
A callback function in JavaScript is a function that is passed as an argument to another function and is executed later, often after an asynchronous operation completes or when a specific event occurs. It allows you to control the flow of your code by defining what should happen once a certain task is finished.
Real life example of callback function – Retrieving data from a server, User Authentication, Form validation, File Reading, Animation Sequencing, Database Operations, etc.
Here’s a simple example:
function doSomethingAsync(callback) {
// Simulating an asynchronous operation (e.g., fetching data)
setTimeout(function() {
console.log("Task completed!");
// Calling the callback function
callback();
}, 1000);
}
// Using a callback function
doSomethingAsync(function() {
console.log("Callback executed!");
});
JavaScript- In this example, ‘doSomethingAsync‘ is a function that simulates an asynchronous operation.
- It takes a callback function as an argument and executes it after the asynchronous task (in this case, a ‘setTimeout‘).
- Callback functions are crucial for handling asynchronous operations, making your code more flexible and responsive.
18. Explain the concept of function scope and block scope.
Function Scope:
Function scope refers to the visibility or accessibility of variables within a function. Variables declared inside a function are local to that function and not accessible outside of it.
For Example:
function exampleFunction() {
var localVar = "I am local";
console.log(localVar); // Accessible inside the function
}
// Uncommenting the line below would result in an error
// console.log(localVar); // Not accessible outside the function
JavaScriptBlock Scope:
Block scope refers to the visibility or accessibility of variables within a block of code, typically denoted by curly braces ‘{}‘.
Variables declared with ‘let‘ and ‘const‘ are block-scoped, meaning they are only accessible within the block where they are defined.
For Example:
if (true) {
let blockVar = "I am block-scoped";
console.log(blockVar); // Accessible inside the block
}
// Uncommenting the line below would result in an error
// console.log(blockVar); // Not accessible outside the block
JavaScript19. Describe the differences between function declarations and function expressions.
Function Declaration:
- Suitable for scenarios where you need the function to be available throughout the entire scope.
- It is a way to define a named function with a specified set of parameters and a function body.
- Function declarations are hoisted and Can be called before the declaration.
Function Declaration Syntax:
function functionName(parameters) {
// Function body
// Code here
}
JavaScriptExample:
sayHello(); // This works even before the function declaration
function sayHello() {
console.log("Hello!");
}
JavaScriptFunction Expressions:
- It is a way to create a function and assign it to a variable or pass it as an argument to another function.
- Function expressions are not hoisted. You can only call the function after the expression has been assigned.
- Useful in scenarios where you need a function as part of an expression (e.g., assigning to a variable or passing as an argument).
- Provides more control over when and where the function is defined and used.
Function Expressions Syntax:
var functionName = function(parameters) {
// Function body
// Code here
};
JavaScriptExample:
// Uncommenting the line below would result in an error
// sayHello(); // This doesn't work here
var sayHello = function() {
console.log("Hello!");
};
sayHello(); // Now the function can be called
JavaScript20. What is call() and apply() methods?
The call() and apply() methods in JavaScript are used to invoke a function with a specified value for ‘this‘ and potentially pass arguments to the function. They are helpful when you want to explicitly set the context (the value of “this”) for a function execution.
call() Method:
The call() method is used to invoke a function with a specified “this” value and individual arguments.
Example:
function greet(message) {
console.log(`${message}, ${this.name}!`);
}
const person = { name: 'John' };
greet.call(person, 'Hello');
// Output: Hello, John!
JavaScriptapply() Method:
The apply() method is similar to call() but takes arguments as an array.
Example:
function introduce(greeting, age) {
console.log(`${greeting}, I'm ${this.name}, and I'm ${age} years old.`);
}
const person = { name: 'Alice' };
introduce.apply(person, ['Hi', 25]);
// Output: Hi, I'm Alice, and I'm 25 years old.
JavaScript21. Explained bind() Method.
The ‘bind()’ method in JavaScript is used to create a new function with a specified ‘this’ value and, optionally, a set of initial arguments. Unlike ‘call()’ and ‘apply()’, ‘bind()’ does not immediately invoke the function; instead, it returns a new function that can be invoked later.
Syntax:
const newFunction = originalFunction.bind(thisArg[, arg1[, arg2[, ...]]]);
JavaScript- ‘originalFunction’: The function for which you want to set the ‘this’ value.
- ‘thisArg’: The value to be passed as the ‘this’ value when the new function is invoked.
- ‘arg1, arg2, …‘: Optional arguments that are prepended to the arguments passed to the new function when it is invoked.
Example:
const person = {
name: 'Alice',
greet: function(greeting) {
console.log(`${greeting}, ${this.name}!`);
}
};
const greetForAlice = person.greet.bind(person, 'Hello');
greetForAlice();
// Output: Hello, Alice!
JavaScriptIn this example,
bind() is used to create a new function greetForAlice that has its ‘this’ value set to the ‘person’ object and a pre-defined greeting of ‘Hello’. When greetForAlice() is invoked, it prints “Hello, Alice!”.
Intermediate JavaScript Interview Questions and Answers 2024 (Suitable for Both Fresher and Experienced)
22. Explain closures in JavaScript?
A closure is a combination of a function and the lexical (surrounding) environment within which that function was declared. It allows the function to retain access to variables from its outer scope even after the outer function has finished executing.
Creating Closures:
Closures are created when a function is defined inside another function, and the inner function captures and remembers the variables from the outer function.
function outerFunction(x) {
// Inner function is a closure, capturing 'x'
function innerFunction(y) {
return x + y;
}
return innerFunction;
}
const closureExample = outerFunction(10);
console.log(closureExample(5)); // Outputs: 15
JavaScriptIn this example, ‘innerFunction’ is a closure because it retains access to the ‘x’ variable even after ‘outerFunction’ has completed.
23. What is the purpose of closures in JavaScript?
Closures in JavaScript serve several important purposes, contributing to the flexibility and power of the language. Here are the key purposes of closures:
- Encapsulation:
Closures allow the bundling of variables and functions into a self-contained unit. The inner function has access to the outer function’s variables even after the outer function has finished executing. This helps in encapsulating and protecting data, preventing unintended modifications from outside.
- Data Privacy and Protection:
Closures provide a mechanism for creating private variables. Variables defined in the outer function are not directly accessible from outside, but the inner function can still access and modify them. This helps in creating modules with hidden implementation details.
- Function Factory:
Closures enable the creation of functions dynamically. A function inside another function can be returned and assigned to a variable, effectively creating a function factory. This is useful for generating functions with specific behaviors based on the context in which they are created.
- Callback Functions:
Closures are commonly used in asynchronous operations and event handling. Callback functions that capture the surrounding state and context rely on closures. This ensures that the callback function has access to the relevant variables even after the outer function has completed its execution.
- Partial Application and Currying:
Closures enable techniques like partial application and currying, where a function is partially applied with some arguments, returning a new function with the remaining arguments. This is useful for creating more flexible and reusable functions.
- Maintaining State:
Closures help in maintaining state between function calls. The inner function retains access to the variables of the outer function, allowing the preservation of state across multiple invocations.
24. What is a pure function?
A pure function in programming is a function that, given the same input, will always return the same output and has no side effects.
The key characteristics of a pure function are:
Determinism: The output of a pure function is entirely determined by its input parameters. For the same set of inputs, a pure function will always produce the same result.
No Side Effects: A pure function does not modify any state outside its own scope. It doesn’t change global variables, perform I/O operations, or alter any external state. Its impact is limited to the computation and return of a value.
Referential Transparency: The function’s output depends solely on its input parameters, without considering any external state. It can be replaced with its result without affecting the program’s behavior.
For Example:
// Pure Function
function square(x) {
return x * x;
}
// Impure Function
let result = 0;
function squareImpure(x) {
result = x * x;
return result;
}
JavaScript25. Explain the concept of function currying.
Function currying is like breaking down a complex task into simpler steps. Instead of dealing with all the information at once, you process one piece at a time. In programming, it means transforming a function that takes many inputs into a series of functions, each handling one input. This makes things more flexible and modular.
Definition:
Function currying is a technique in functional programming where a function is transformed into a sequence of functions, each taking a single argument. The process of breaking down a function that takes multiple arguments into a series of functions that take one argument at a time is called currying.
Example 1: Non-currying function technique
// Non-currying technique
function add(a, b, c) {
return a + b + c;
}
const result1 = add(1, 2, 3); // Output: 6
JavaScriptExample 2: Currying function technique
// Currying technique
function curryAdd(a) {
return function(b) {
return function(c) {
return a + b + c;
};
};
}
const curriedAdd = curryAdd(1)(2)(3);
const result2 = curriedAdd; // Output: 6
JavaScript- ‘curryAdd’ is a curried version of the ‘add’ function.
- It takes the first argument ‘a’ and returns a function.
- The returned function takes the second argument ‘b’ and returns another function.
- The final returned function takes the third argument ‘c’ and performs the multiplication.
26. Explain the difference between ‘map’ and ‘forEach’.
map():
The ‘map()’ is a higher-order array method used to create a new array by applying a provided function to each element of the original array.
Syntax:
map is a higher-order array method used to create a new array by applying a provided function to each element of the originaconst newArray = originalArray.map(function(element, index, array) {
// Transformation logic here
return transformedElement;
});
JavaScriptforEach():
The ‘forEach()’ is a method in JavaScript that is used to iterate over the elements of an array. It executes a provided function once for each element in the array, in ascending order(natural order).
Syntax:
array.forEach(function(currentValue, index, array) {
// Your code here
}, thisArg);
JavaScriptDifferences between forEach() and map() methods
forEach() | map() |
The forEach() method does not return a new array based on the given array. | The map() method returns an entirely new array. |
The forEach() method returns “undefined“. | The map() method returns the newly created array according to the provided callback function. |
The forEach() method doesn’t return anything hence the method chaining technique cannot be applied here. | With the map() method, we can chain other methods like, reduce(), sort(), etc. |
It is not executed for empty elements. | It does not change the original array. |
27. What is the difference between arguments and parameters?
The terms “arguments” and “parameters” are often used interchangeably, but they have distinct meanings in the context of programming, especially in JavaScript.
Parameters | Arguments |
Parameters are variables that are used in a function declaration or definition. | Arguments are the actual values that are passed when the function is invoked or initialized. |
They are part of the function signature, which appears in parentheses. | They are the values provided inside the parentheses when calling the function. |
Parameters act as placeholders for values that are expected by the function. | Arguments provide values for the parameters defined in the function. |
Example: function add(a, b) { return a + b; } | Example: let result = add(5, 3); |
28. What is the difference between ‘for…of‘ and ‘for…in’ loop?
In JavaScript, ‘for…of’ and ‘for…in’ are both loop constructs, but they are used for different purpose and iterate over different types of values.
for…of:
Primarily used for iterating over objects like arrays, strings, maps, sets, etc. Provides direct access to the values of the iterable, rather than their indices.
Example:
let fruits = ['apple', 'banana', 'orange'];
for (let fruit of fruits) {
console.log(fruit); // Output: apple, banana, orange
}
JavaScriptfor…in:
It is used to go through all the visible properties of an object. This includes not only the properties that the object directly has but also those it inherits from its parent objects.
Example:
let person = { name: 'John', age: 30 };
for (let key in person) {
console.log(key); // Output: name, age
console.log(person[key]); // Output: John, 30
}
JavaScript29. What is the purpose of the filter() method?
The filter method in JavaScript is used to create a new array by selectively including elements from an existing array based on a specified condition. It does not modify the original array but instead returns a fresh array containing only the elements that meet the criteria defined in the provided function. This is particularly useful when you want to extract or manipulate data based on certain conditions without altering the original data structure.
Example:
let originalArray = [1, 2, 3, 4, 5];
let newArray = originalArray.filter(element => element > 2);
console.log(newArray); // Output: [3, 4, 5]
JavaScript30. Explain reduce() method?
The reduce method in JavaScript is like a helper that helps you combine all the numbers in a list to get one single result. It’s like adding each number to a list or doing something with it and keeping track of the total as you go. You can use it for things like finding the total sum of numbers, getting the highest or lowest value, or performing more complex calculations with an array of data.
Read more about reduce() here.
Syntax:
array.reduce(function(accumulator, currentValue, currentIndex, array) {
// Your logic here
}, initialValue);
JavaScriptExample:
let numbers = [1, 2, 3, 4, 5];
let totalSum = numbers.reduce(function(result, num) {
return result + num;
});
console.log(totalSum); // Output: 15
JavaScript31. What is the use of the hasOwnProperty method?
The hasOwnProperty method in JavaScript is a tool that allows us to check if an object directly contains a specific property. It helps us avoid potential issues when working with objects, especially in scenarios where properties might be inherited from prototypes.
This method returns a boolean value, true if the object has the specified property, and false if it doesn’t. It’s a handy way to distinguish between properties that belong directly to an object and those that may come from the object’s prototype chain.
Example:
let person = {
name: 'John',
age: 25,
city: 'Example City'
};
console.log(person.hasOwnProperty('name')); // Output: true
console.log(person.hasOwnProperty('gender')); // Output: false
JavaScript32. What is object cloning?
Object cloning in JavaScript is the process of creating a duplicate or copy of an existing object. When we clone an object, we aim to create a new object with the same properties and values as the original. It is important to understand that in JavaScript, Objects are reference types, which means that when you assign an object to a variable or pass it as an argument, you are dealing with a reference to the original object rather than an independent copy.
There are different ways to clone an object in JavaScript.
1. Shallow Cloning:
Shallow cloning creates a new object and copies the top-level properties of the original object. However, if the properties are object themselves, they still reference the same objects in both the original and cloned object. Shallow cloning methods include ‘Object.assign()’ and the spread operator (‘{…}’).
let originalObject = { name: 'John', details: { city: 'Example City' } };
// Shallow cloning with Object.assign()
let shallowCloneAssign = Object.assign({}, originalObject);
// Shallow cloning with spread operator
let shallowCloneSpread = { ...originalObject };
JavaScript2. Deep Cloning:
Deep cloning creates a completely independent copy of the original object, including all nested object. Changes made to nested objects in the clone do not affect the original object. A common method for deep cloning is using ‘JSON.parse(JSON.stringify())’, but it has limitations and may not work well with objects containing functions or special values.
let originalObject = { name: 'John', details: { city: 'Example City' } };
// Deep cloning with JSON.parse(JSON.stringify())
let deepClone = JSON.parse(JSON.stringify(originalObject));
JavaScript33. What are template literals in ES6?
Template literals were introduced in ES6 to provide a more flexible and concise way of working with strings. They allow us to embed variables and expressions directly into strings using ${}. This feature is known as string interpolation which facilitates the creation of dynamic strings.
Additionally, template literals support multiline strings without escape characters, increasing code readability. They are a standard and widely accepted feature in modern JavaScript to improve string handling in our code.
let name = 'John';
let age = 25;
// String literal with variable interpolation
let greeting = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(greeting);
JavaScript34. Explain the Arrow function in ES6.
An arrow function (() =>) is a concise method introduced in ES6 for writing functions in JavaScript. This syntax is designed to make our code more compact and readable, particularly for short, one-line functions.
Arrow functions are essentially anonymous functions, means they don’t have a name. but, they are commonly assigned to variables for reuse in code. Arrow functions play an important role in improving the structure and readability of JavaScript code.
Example:
// Arrow function for multiplication
let multiply = (a, b) => a * b;
// Using the arrow function
console.log(multiply(5, 3)); // Output: 15
JavaScript35. What is a destructuring assignment in ES6?
Destructuring assignment in ES6 is a feature that allows us to extract values from arrays or properties from objects and assign them to variables more concisely.
In array destructuring, we use square brackets [] to unpack values, while in object destructuring, we use curly braces {} to extract properties.
This feature is beneficial for making code more readable, reducing the need for temporary variables, and offering a cleaner syntax for handling array and object values.
Example:
// Example data representing a person
let personData = ['John', 25, 'Example City'];
// Array destructuring to assign values to variables
let [name, age, city] = personData;
// Object representing additional information about the person
let additionalInfo = { occupation: 'Engineer', hobbies: ['Reading', 'Coding'] };
// Object destructuring to assign properties to variables
let { occupation, hobbies } = additionalInfo;
// Outputting the extracted values
console.log(`Name: ${name}`);
console.log(`Age: ${age}`);
console.log(`City: ${city}`);
console.log(`Occupation: ${occupation}`);
console.log(`Hobbies: ${hobbies.join(', ')}`);
JavaScript36. What is the event loop in JavaScript?
The event loop in JavaScript is a runtime mechanism that manages the execution flow of code in a single-threaded environment. This enables the handling of asynchronous operations and events without blocking the main execution thread.
The event loop continuously checks for tasks or events in an event queue, moving them to the call stack for execution when the stack is empty. This process ensures that JavaScript remains responsive, efficiently managing both synchronous and asynchronous tasks in a non-blocking manner.
Learn About Event Loop in JS – Recommended Resources:
37. Explain the purpose of promises in JavaScript.
A Promise in JavaScript is an object that represents the eventual completion or failure of an asynchronous operation and its resulting value. Promises enhance code readability by providing a cleaner syntax for handling asynchronous tasks, reducing the complexity associated with callbacks. They allow us to better organize asynchronous code, making it more maintainable and reducing the risk of callback hell.
They also facilitate error handling through the use of the .catch() method. Additionally, promises support chaining and sequencing of asynchronous tasks, leading to more readable and modular code. The integration of promises with async/await further enhances the development of asynchronous code that closely resembles synchronous code, improving overall code structure and maintainability.
Example:
// Example function that returns a promise
const fetchData = () => {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation (e.g., fetching data)
const success = true;
// Simulating a delay of 1000 milliseconds
setTimeout(() => {
// Check if the operation was successful
if (success) {
// Resolve the promise with the data
resolve({ message: "Data fetched successfully" });
} else {
// Reject the promise with an error
reject(new Error("Failed to fetch data"));
}
}, 1000);
});
};
// Using the promise with .then() and .catch()
fetchData()
.then((result) => {
console.log(result.message);
})
.catch((error) => {
console.error(error.message);
});
JavaScript38. What is async/await and how does it work
Async/await is a feature in JavaScript that simplifies asynchronous code. When a function is declared as ‘async‘, it indicates that it will perform asynchronous operations and will always return a Promise. The ‘await‘ keyword is used inside an ‘async’ function to pause the execution until a Promise is resolved. This allows us to write asynchronous code in a more sequential and straightforward manner
It simplifies handling Promises and error management using try-catch blocks. This feature enhances code readability and helps avoid callback hell by providing a cleaner and more structured way to work with asynchronous operations.
39. What is the DOM in JavaScript?
DOM refers to the Document Object Model, an interface that represents the structure of an HTML or XML document as a tree-like object. It allows JavaScript to dynamically access and manipulate the content, structure, and style of a web page.
Learn More About DOM here.
40. Explain the concept of event bubbling and capturing.
Event bubbling and capturing are two phases of event propagation in the Document Object Model(DOM) when an event occurs.
Event bubbling is the process where the event starts from the target element that triggered the event and bubbles up to the root of the document. During bubbling, the innermost element’s event handler is executed first, followed by its parent, and so on until reaching the document root.
In event capturing, or trickling it’s the opposite process, the event starts at the root and moves down to the target element. During this, the event handler on the outermost ancestor is executed first, and followed by its descendants until it reaches to the target element.
41. What is Ajax?
AJAX, or Asynchronous JavaScript and XML, is a powerful web development technique that enables the asynchronous exchange of data between a web browser and a server. Its key features play a crucial role in creating more dynamic and responsive web applications.
One significant application of AJAX lies in dynamic content loading. Instead of the conventional approach of reloading the entire web page, AJAX empowers us to selectively update specific parts of the page with fresh data from the server. This not only enhances user experience but also ensures faster and smoother interactions.
To achieve this, AJAX heavily relies on JavaScript to make asynchronous requests. This means that data can be exchanged between the client and the server in the background, without interrupting the user’s experience on the web page.
In essence, AJAX serves as a foundational tool for developing modern and interactive web applications. It provides a seamless and efficient mechanism for data exchange between the client and the server, ultimately elevating the overall user experience.
42. How do you make an HTTP request in JavaScript?
In JavaScript, making HTTP requests is crucial for fetching data from servers and updating the content of web applications. There are several ways to make HTTP requests, each catering to different needs. But there are two common methos for achieving this: using the traditional ‘XMLHttpRequest‘ object and the more modern ‘Fetch API‘.
With ‘XMLHttpRequest’, we create an instance, configure it, set up event listeners to handle responses, and send the request. This approach has been widely used in the past but may involve more boilerplate code.
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/data', true);
xhr.onload = function () {
if (xhr.status === 200) {
console.log(xhr.responseText);
} else {
console.error('Error:', xhr.statusText);
}
};
xhr.send();
JavaScriptOn the other hand, the ‘Fetch API’ is a newer and more modern approach. It simplifies the process with a cleaner syntax and a Promise-based structure, making asynchronous operations more straightforward.
fetch('https://example.com/api/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
JavaScript43. What is CORS?
CORS (Cross-Origin Resource Sharing) is a security implemented by browsers to control how web pages in one domain can request and interact with resources from another domain. This security measure is meant to prevent potentially harmful cross-origin requests.
Handling CORS in JavaScript primarily involves server-side configurations. The server must include the proper headers, such as ‘Access-Control-Allow-Origin’, to specify which domains are allowed access.
44. How do you handle CORS in JavaScript?
Handling CORS in JavaScript is crucial for ensuring secure and seamless cross-origin interactions. I approach this challenge completely, focusing on both server-side configurations and client-side strategies.
Firstly, I emphasize robust server-side configurations by implementing necessary CORS headers, such as ‘Access- Control-Allow-Origin’ and, if needed, ‘Access-Control-Allow-Methods’ and ‘Access-Control-Allow-Headers’. This ensures that the server explicitly defines which origins, method, and headers are allowed, providing a secure foundation for cross-origin requests.
If I’m working with a server-side framework like Express.js, I take advantage of middleware like ‘cors’ for simplified CORS configuration.
For scenarios where the server lacks native CORS support, especially with GET request, I consider JSONP.
Additionally, I explore the option of a proxy server on the same domain. This approach allows for the forwarding of request, bypassing CORS restrictions and facilitating secure cross-origin communication.
By adopting a combination of these server-side and client-side strategies, I ensure a robust and flexible CORS handling mechanism.
45. What is the purpose of the try, catch, and finally blocks?
‘try‘ statement defines a block of code to test for errors during execution. If an error occurs, the ‘catch‘ statement allows us to define a block of code to handle and manage the error smoothly. The ‘finally‘ block provides a reliable way to handle cleanup logic and ensures that critical operations are performed. This block executes regardless of whether an exception occurred or not.
Example:
function exampleFunction() {
try {
// Code that might throw an exception
throw new Error("An example error");
} catch (error) {
// Handling the exception
console.error(`Caught an error: ${error.message}`);
} finally {
// Code that always runs, whether there was an exception or not
console.log("This code always runs");
}
}
// Example usage
exampleFunction();
JavaScript46. What is the purpose of testing frameworks like Jasmine or Mocha?
The basic purpose of testing frameworks like Jasmine or Mocha is for software development and maintaining robust and reliable JavaScript code. These frameworks provide a structured and efficient way to create and execute tests, helping to ensure the correctness and functionality of your code during the development process.
Testing frameworks like Jasmine or Mocha provide features like test organization, assertions, and reporting, making it easier for developers to write, run, and manage tests effectively. Using testing frameworks in software development helps to catch and resolve issues early, increase code quality, and achieve a more seamless development workflow.
47. How do you handle cross-browser compatibility issues?
Handling cross-browser compatibility issues in JavaScript is crucial for ensuring a consistent user experience across different browsers. Here are some key strategies.
1. Feature Detection over Browser Detection:
I prioritize feature detection using methods like typeof or in to check if a particular feature is supported. This approach is more robust than browser detection as it focuses on capabilities rather than specific browser versions.
2. Polyfills for Missing Features:
I utilize polyfills for features not supported in certain browsers. This involves including additional JavaScript that provides the missing functionality, ensuring a uniform experience across browsers.
3. CSS Resets and Normalization:
To address inconsistencies in default styles, I employ CSS resets or normalizations. This helps establish a consistent baseline for styling, preventing unexpected variations across browsers.
4. Regular Cross-Browser Testing:
I conduct thorough testing on multiple browsers, including popular choices like Chrome, Firefox, Safari, and Edge. This proactive approach helps identify and rectify issues specific to each browser.
5. Cross-Browser Testing Tools:
I leverage cross-browser testing tools such as BrowserStack or Sauce Labs to simulate user experiences across various browsers and devices. These tools help catch potential issues before deployment.
6. Responsive Design Principles:
I adhere to responsive design principles, ensuring that web applications adapt gracefully to different screen sizes and resolutions. This contributes to a seamless user experience on diverse devices.
48. How do you handle cross-browser compatibility issues?
Task runners like Grunt or Gulp play a pivotal role in automating various repetitive tasks during the development process. Their primary purposes include:
1. Automation of Repetitive Tasks:
Task runners automate repetitive tasks such as minification, compilation, and bundling of code, making the development workflow more efficient.
2. Code Optimization:
Task runners enable code optimization by automating tasks like minification and concatenation, resulting in smaller file sizes and improved website performance.
3. Live Reloading and Development Servers:
Some task runners facilitate live reloading, automatically refreshing the browser when changes are made. They may also provide development servers for local testing.
4. Build Process Simplification:
They simplify the build process by allowing developers to define and execute a series of tasks in a specific order.
49. How do you prevent XSS attacks in JavaScript?
Prevent XSS attacks is important for the maintaining the security of web applications. You can follow following approach to prevent XSS attack.
1. Input Validation:
Fully validate and sanitize all user input on both client and server sides. This helps ensure that only necessary and secure data is processed.
2. Output Encoding:
Before rendering user-generated content, be sure to apply the correct output encoding. This involves converting special characters into HTML elements, preventing any injected scripts from being executed.
3. Content Security Policy (CSP):
Implementing a robust CSP is essential. It defines trusted sources for content, restricting the execution of script from unauthorized locations and providing an additional layer of security.
4. Avoidance of Inline JavaScript:
Minimizing the use of inline JavaScript is a good practice. If unavoidable, employing nonce or hash attributes within CSP can control the execution of inline scripts.
5. Regular Security Audits:
Conducting regular security audits is part of our development process. This involves both automated tools and manual reviews to identify and reduce the risk of a security vulnerability.
50. What is Content Security Policy (CSP)?
Content Security Policy (CSP) is a security standard implemented by web browsers to reduce the risks associated with certain types of web-based attacks, particularly cross-site scripting (XSS) attacks. This is an additional layer of security that helps prevent the execution of malicious scripts on web pages.
Developers can specify trusted sources for various types of content, such as scripts, stylesheets, images, fonts and more. This helps ensure that only approved content is loaded and executed on the web pages.
CSP enables the restriction of inline scripts, which are scripts embedded directly into HTML documents. Developers can define policies that allow only certain scripts to be executed from trusted sources, reducing the risk of XSS attacks.
51. Explain techniques to improve JavaScript performance.
Improving JavaScript performance is important for building fast and responsive web applications. Here are some key techniques:
- Minify and Compress JavaScript code
- Bundle Files to reduce HTTP request
- Optimize Loops
- DOM Optimization
- Lazy Loading
- Memory Management
- Profiling
Advanced JavaScript Interview Questions and Answers for Experienced (2024)
52. Describe the Virtual DOM in React.
Virtual DOM is a performance optimization technique in React. Instead of directly manipulating a web page’s actual Document Object Model (DOM), React maintains a lightweight, in-memory representation known as a virtual DOM.
The Virtual DOM is the virtual representation of Real DOM, which is just like a blueprint of a machine, It can make changes in the blueprint, but those changes will not directly apply to the machine. React update the changes in virtual DOM first and then it syncs with real DOM. The Virtual DOM enhances performance which makes it faster.
53. What is JSX in React?
JSX stands for JavaScript XML. It is a syntax extension that allows you to write code in a syntax similar to XML/HTML inside a JavaScript file. Browsers don’t understand JSX directly, so we need to convert it into regular JavaScript using a transpilation process. JSX allow us to express React elements more clearly, making it easier for us to understand the component’s structure.